-- Query to delete all the Stored Procedure, Function (Scalar and Table Value Function) and User Defined Tables within the database
DECLARE @Cnt as INTEGER
DECLARE @SPName as varchar(100)
DECLARE @FuncName as varchar(100)
DECLARE @TableName as varchar(100)
DECLARE @Qry as varchar(200)
-- Stored Procedure
select @Cnt = Count(id) from sysobjects where type = 'p'
WHILE (@Cnt > 0)
BEGIN
SELECT @SPName = name From
(SELECT row_number() over (order by name) as rownumber, name from Sysobjects where type = 'p') t WHERE rownumber = @Cnt
exec ('DROP Procedure ' + @SPName)
SET @Cnt = @Cnt - 1
END
--Table Valued Function
select @Cnt = Count(id) from sysobjects where type = 'TF'
WHILE (@Cnt > 0)
BEGIN
SELECT @FuncName = name From
(SELECT row_number() over (order by name) as rownumber, name from Sysobjects where type = 'TF') t
WHERE rownumber = @Cnt
exec ('DROP Function ' + @FuncName)
SET @Cnt = @Cnt - 1
END
--Scalar valued Function
select @Cnt = Count(id) from sysobjects where type = 'FN'
WHILE (@Cnt > 0)
BEGIN
SELECT @FuncName = name From
(SELECT row_number() over (order by name) as rownumber, name from Sysobjects where type = 'FN') t
WHERE rownumber = @Cnt
exec ('DROP Function ' + @FuncName)
SET @Cnt = @Cnt - 1
END
-- Tables
SELECT @Cnt = Count(id) from sysobjects where type = 'u'
WHILE (@Cnt > 0)
BEGIN
SELECT @tablename = name FROM
(SELECT row_number() over (order by name) as rownumber, name from Sysobjects where type = 'u') t
WHERE rownumber = @Cnt
--To Delete all the Constraints for the Table
IF(SELECT Count(Constraint_Name) FROM INFORMATION_SCHEMA.TABLE_CONSTRAINTS WHERE table_Name = @tablename) > 0
BEGIN
SELECT @Qry = 'ALTER TABLE ' + @tablename + ' DROP CONSTRAINT ' + CONSTRAINT_NAME
FROM INFORMATION_SCHEMA.TABLE_CONSTRAINTS WHERE table_name = @tablename
EXEC sp_executesql @Qry
END
--To Delete all the Records in the Table
EXEC ('Truncate Table ' + @tablename)
--To Delete the Table
EXEC ('Drop Table ' + @tablename)
SET @Cnt = @Cnt - 1
END
Captions
- Asp.Net (9)
- ASP.Net Tips (1)
- C# (7)
- Computer (1)
- Internet Explorer (5)
- Java Script (11)
- Shortcut Keys (4)
- SQL Programming - Common Mistakes (11)
- Sql Server (22)
- Sql Server Definitions (8)
- Sql Server Test (2)
- SSRS (2)
About Me
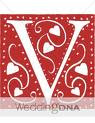
- Vasanth.S
- I'm just normal guy who like to travel, addicted to TV and Computer, Playing Computer Games and Watching Movies and many more. Have lot of idea and quote in life
My Blog List
-
-
Pass data from one page to another using post15 years ago
Blog Archive
-
▼
2009
(63)
-
▼
July
(12)
- Insert into Table with XML Value as Parameter
- Error Handling in Sql Server
- Procedure to find a given Number is Amstrong Numbe...
- Procedure to Upload CSV File to Table
- Basics of Sql Server - 2
- Basics of Sql Server - 1
- Working Hours between two Dates excluding Weekends
- No. of Saturday and Sunday between two Dates
- Getting Column value in a row with comma seperator
- Split the variable based on a demiliter
- Date Parameters
- Delete all Stored Procedure, Function and Tables i...
-
▼
July
(12)